C programming is a foundational language that has stood the test of time in the computer science world. Whether you’re new to programming or looking to solidify your coding skills, mastering C is essential. It is often referred to as the “mother of all languages” because many modern languages like C++, Java, and Python are influenced by its syntax and structure. This article will walk you through the fundamentals of C programming, providing you with the knowledge needed to succeed as a computer science student.
Top Courses in Programing Language
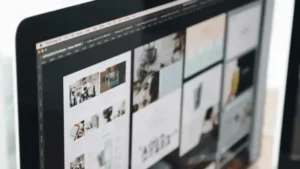
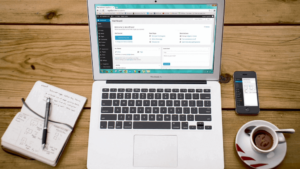
More Courses With Certification
Why Learn C Programming?
C programming is highly versatile and powerful. It gives you deep control over hardware, making it ideal for systems programming, embedded programming, and developing performance-critical applications. Additionally, C is widely used in operating system development, game development, and computational programming. Learning C can also help you better understand concepts such as
Basic Structure of a C Program
A simple C program includes the following components:
- Preprocessor Directives: These are commands that instruct the compiler to include libraries or define constants. For example,
#include
<stdio.h>
includes the standard input-output library.
- Main Function: Every C program must include a main() function, which serves as the entry point. This function serves as the starting point for program execution.
- Statements and Expressions: Inside the main function, students write statements that perform actions, such as printing output or performing calculations.
- Return Statement: The
return 0;
statement indicates that the program has executed successfully.
Data Types and Variables
C supports several data types, including:
- int: for integers
- float: for floating-point numbers
- char: for characters
- double: for double-precision floating-point numbers
Control Structures
Control structures allow students to dictate the flow of their programs. The main types include:
- Conditional Statements:
if
,else if
, andelse
statements enable decision-making in programs.
- Loops: (for, while, and do-while) allow code blocks to be executed repeatedly.
- Switch Statement: This provides a way to execute different parts of code based on the value of a variable.
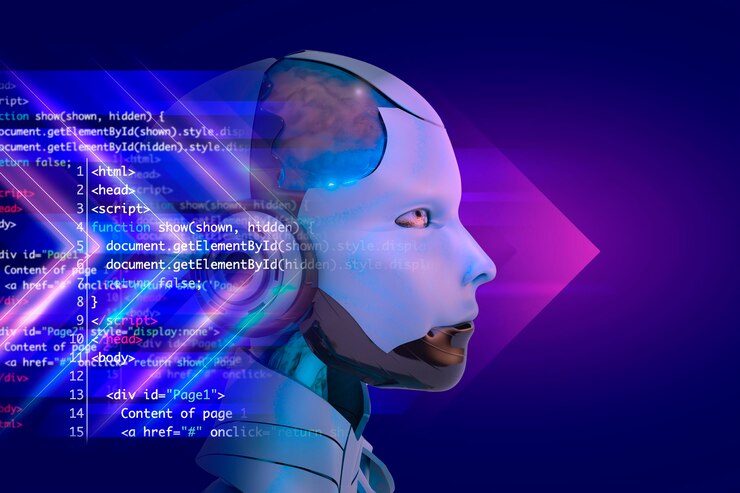
Functions
Functions in C allow you to break down a program into smaller, manageable pieces. Each function serves a specific purpose and can be reused throughout the program. Functions also promote modularity and code reusability, making programs easier to read and debug.
Arrays
Arrays are used to store multiple values of the same type in a single variable. They are crucial when dealing with large amounts of data. In C, arrays are zero-indexed, which means the first element is at index zero.
Pointers
Pointers are one of the most powerful and complex features of C. They save the memory address of a variable. Understanding pointers is essential for dynamic memory allocation, arrays, and functions.
Structures
Structures allow you to arrange many data kinds together. They are useful for creating complex data types that better represent real-world objects.
Memory Management
Unlike higher-level languages, C provides low-level memory access through functions like malloc()
, calloc()
, realloc()
, and free()
. Efficient memory management is critical for improving program performance.
Debugging and Error Handling
As you write more complex programs, debugging becomes a crucial part of development. Common errors in C include syntax errors, logical errors, and runtime errors. It’s essential to develop good debugging skills, which include:
- Reading error messages carefully: They often provide clues about what went wrong.
- Using a debugger: Tools like GDB (GNU Debugger) can help you step through your code and identify issues.
Top Courses in Computer Science Engineering
More Courses With Certification
Best Practices for C Programming
- Comment your code: Use comments to explain complex parts of your code for future reference.
- Write modular code: Break your program into smaller functions to enhance readability and reusability.
- Handle errors gracefully: Use error-checking techniques to handle unexpected inputs and conditions.
- Optimize your code: Focus on writing efficient code, especially when working on performance-critical applications.
Important Announcement – EasyShiksha has now started Online Internship Program “Ab India Sikhega Ghar Se”
FAQs: Frequently Asked Questions
Q1: Is C programming difficult to learn for beginners?
A: While C programming can be challenging at first due to its syntax and concepts like pointers, with regular practice and a clear understanding of the fundamentals, it becomes easier. Starting with simple programs and gradually working your way up will help you master it.
Q2: How does C differ from modern programming languages like Python or Java?
A: C provides more direct control over system resources such as memory and hardware, while higher-level languages like Python or Java offer abstractions to make programming easier and faster. However, C’s low-level capabilities make it ideal for system-level programming.
Q3: What are some common applications of C programming?
A: C is widely used in operating system development (Linux, Windows), embedded systems, game development, and high-performance applications like compilers and databases.
Q4: Do I need to learn C if I want to specialize in web or app development?
A: While C is not directly used for web or app development, learning C builds a strong foundation in programming logic and memory management, which are valuable skills across all programming domains.
Related Article: The Role of Computer Science in Cybersecurity
Get Courses: networking course
Conclusion
Mastering C programming is an essential step for any computer science student. With a solid grasp of the fundamentals, you can build a strong foundation that will serve you well in advanced topics like data structures, algorithms, and systems programming. Regular practice and breaking down complex problems into smaller, manageable parts will help you become proficient in C.
For those looking for structured courses and hands-on experience, EasyShiksha.com provides a range of programming tutorials, including C, to help you on your journey to becoming a skilled developer. Happy coding!