What is State in React?
What is State in React? State is a JavaScript object that stores component’s dynamic data and it enables a component to keep track of changes between renders. Because state is dynamic, it is reserved only for interactivity so you don’t use it for static React projects.
Components defined as classes have some additional features. Local state is exactly that: a feature available only to classes. State can only be used within a class and usually the only place where you can assign this.state is the constructor.
Important Announcement – EasyShiksha has now started Online Internship Program “Ab India Sikhega Ghar Se”
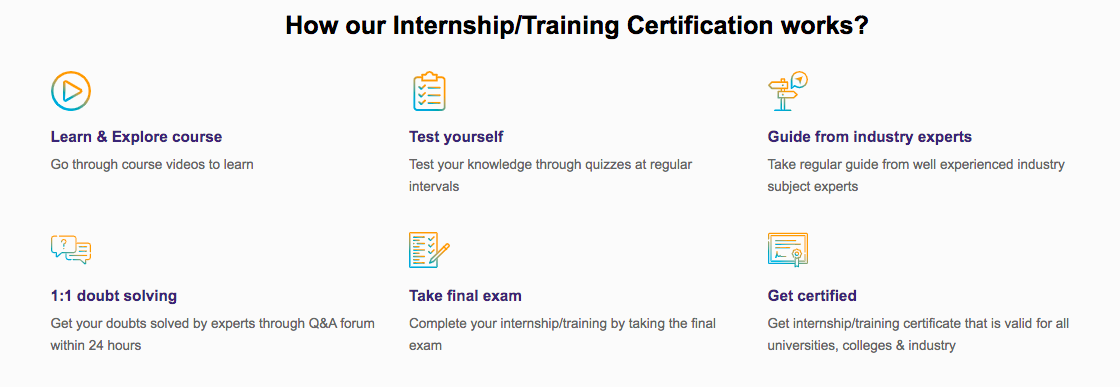
class Greeting extends React.Component {
constructor() {
super();
this.state = {
name: ‘John Smith’
}
}
render() {
return<h1>Hello, my name is { this.state.name }</h1>;
}
}
class Greeting extends React.Component {
state = {
name: ‘John Smith’
}
}
render() {
return <h1>Hello, my name is { this.state.name }</h1>;
}
}
State Updates May Be Asynchronous
What is State in React? React may batch multiple setState() calls into a single update for performance. Calls to setState are asynchronous when they are inside event handlers and that when you don’t rely on this.state to reflect the new value immediately after calling setState.
Top Courses in Software Engineering
More Courses With Certification
incrementCount() {
// Note: this will *not* work as intended.
this.setState({count: this.state.count + 1});
}
handleSomething() {
// Let’s say `this.state.count` starts at 0.
this.incrementCount();
this.incrementCount();
this.incrementCount();
// When React re-renders the component, `this.state.count` will be 1, but you expected 3.
// This is because `incrementCount()` function above reads from `this.state.count`,
// but React doesn’t update `this.state.count` until the component is re-rendered.
// So `incrementCount()` ends up reading `this.state.count` as 0 every time, and sets it to 1.
To fix it, use a second form of setState that accepts a function rather than an object to ensure the call always uses the most updated version of state. i hope now it’s clear to you What is State in React?
Passing an update function allows you to access the current state value inside the updater. Since setState calls are batched, this lets you chain updates and ensure they build on top of each other instead of conflicting:
incrementCount() {
this.setState((state) => {
// Important: read `state` instead of `this.state` when updating.
return {count: state.count + 1}
});
}
handleSomething() {
// Let’s say `this.state.count` starts at 0.
this.incrementCount();
this.incrementCount();
this.incrementCount();
// If you read `this.state.count` now, it would still be 0.
// But when React re-renders the component, it will be 3.
}
Also you can pass props as a second argument at the time the update is applied:
// Correct
this.setState((state, props) => ({
counter: state.counter + props.increment
}));
State Updates are Merged
When you call setState(), React merges the object you provide into the current state.
For instance, your state may contain several independent variables:
constructor(props) {
super(props);
this.state = {
posts: [],
comments: []
};
}
Then you can update them independently with separate setState() calls:
componentDidMount() {
fetchPosts().then(response => {
this.setState({
posts: response.posts
});
});
fetchComments().then(response => {
this.setState({
comments: response.comments
});
});
}
The merging is shallow, so this.setState({comments}) leaves this.state.posts intact, but completely replaces this.state.comments.
You might be asking what is ComponentDidMount() and how it is used. It is a method that is invoked immediately after a component is mounted (inserted into the tree). Initialization that requires DOM nodes should go here. So if you need to load data from a remote endpoint (API), this is a good place to instantiate the network request.
Empower your team. Lead the industry
Get a subscription to a library of online courses and digital learning tools for your organization with EasyShiksha
Request NowWhat is State in React? As you can see in the above example the properties for this.state are now independent and separately added this.setState , passed an object to each property and surrounded them by ComponentDidMount() where they will be added to the DOM tree after we get the response from the fetchPosts() and fetchComments().
I hope you like this blog, What is State in React? To learn more visit HawksCode and Easyshiksha.
ALSO READ: 3-indias-engineering-graduates-land-high-quality-tech-jobs
Get Course: Digital-Marketing-Fundamentals-SEO